Hey guys, what is up. In this particular post, we will be discussing some more operations that are performed in two-dimensional arrays. These are transpose of the matrix in c, the product of two matrices, and displaying of any part of a matrix.
Updated on 1/10/2021
KEY POINTS
TOPICS TO BE COVERED:
Operations on two-dimensional arrays:
- Transpose of the matrix
- Product of two arrays
- Displaying part of a matrix
Transpose of the matrix:
I am pretty sure that you might have heard about this word in mathematics. In chapter matrix and determinants. If you haven’t heard about this word then you will understand its meaning here. Transpose of a matrix is to change rows of a matrix by its columns. Changing rows means to interchange the elements of row by column elements. Row one elements will be interchanged with column only. In the same way row, two elements will be interchanged using columns two elements, and so on. Moreover, these operations can only be performed in a square matrix of order n×n. Moreover, n should be a finite number.
Working of the transpose of the matrix program:
First of all, we will take the parameters of the matrix. Parameter of a matrix means to take the number of rows and columns of a matrix. We will do transpose a matrix with the help of a second array. Also, we will store all the values after transposing them to a second array that will have dimensions the same as that of the first array. Then we will run two loops because it has rows and columns. First, the loop will be for rows. Moreover, second for columns. Both the loops will start from zero to the maximum number of rows and columns minus one respectively.
PROGRAM:
#include<stdio.h>
void main()
{
int i,j,m,n;
int A[10][10], B[10][10];
printf(“ENTER DIMENSIONS OF MATRIX \n”);
printf(“ENTER NUMBERS OF ROWS OF MATRIX= “);
scanf(“%d”,&m);
printf(“ENTER NUMBER OF COLUMNS OF MATRIX= “);
scanf(“%d”,&n);
printf(“ENTER ELEMENTS OF MATRIX\n”);
for(i=0;i<m;i++)
{
for(j=0;j<n;j++)
{
printf(“A[%d][%d]=”,i,j);
scanf(“%d”,&A[i][j]);
}
printf(“\n”);
}
printf(“\nARRAY BEFORE TRANSPOSE\n”);
for(i=0;i<m;i++)
{
for(j=0;j<n;j++)
{
printf(“%d \t”,A[i][j]);
}
printf(“\n”);
}
//CODE OF TRANSPOSE
for(i=0;i<m;i++)
{
for(j=0;j<n;j++)
{
B[i][j]=A[j][i];
}
}
printf(“\nARRAY AFTER TRANSPOSE\n”);
for(i=0;i<m;i++)
{
for(j=0;j<n;j++)
{
printf(“%d \t”,B[i][j]);
}
printf(“\n”);
}
}

Product of two arrays in c:
You might have heard about this in your maths or computer course in class 11th and 12th according to the Indian education system. The product of two matrices is a lit bit difficult and confusing. Everyone thinks it is normal multiplication but no it is a combination of multiplication and addition.
A necessary condition in the multiplication of two arrays:
The number of columns of the first array should be equal to the amount of rows of the second matrix then only the product is allowed.
Working:
In this, we will use three arrays. The third array is used to store the product of two. In this, we will use three loops. First for accessing row elements. Second for accessing column elements. The final loop will be for adding the elements.
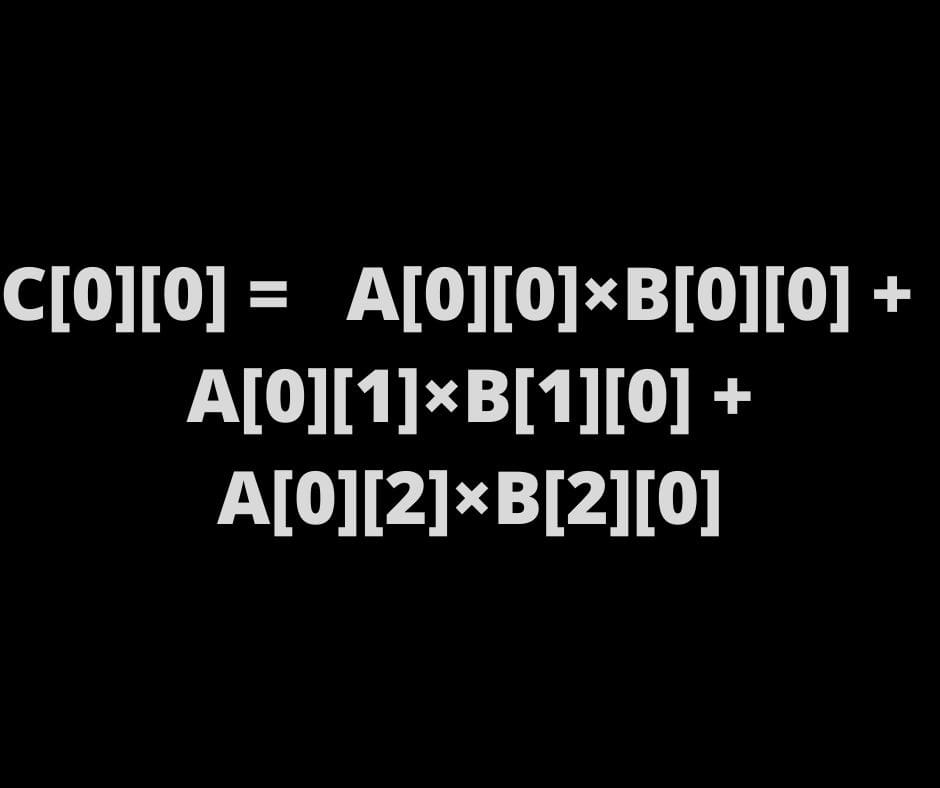
#include <stdio.h>
int main()
{
int m, n, p, q, c, d, k, sum = 0;
int first_matrix[10][10], second_matrix[10][10], multiply[10][10];
printf(“Enter number of rows and columns of first matrix\n”);
scanf(“%d%d”, &m, &n);
printf(“Enter elements of first matrix\n”);
for (c = 0; c < m; c++)
for (d = 0; d < n; d++)
scanf(“%d”, &first_matrix[c][d]);
printf(“Enter number of rows and columns of second matrix\n”);
scanf(“%d%d”, &p, &q);
if (n != p)
printf(“The multiplication isn’t possible.\n”);
else
{
printf(“Enter elements of second matrix\n”);
for (c = 0; c < p; c++)
for (d = 0; d < q; d++)
scanf(“%d”, &second_matrix[c][d]);
for (c = 0; c < m; c++) {
for (d = 0; d < q; d++) {
for (k = 0; k < p; k++) {
sum = sum + first_matrix[c][k]*second_matrix[k][d];
}
multiply[c][d] = sum;
sum = 0;
}
}
printf(“Product of the matrices:\n”);
for (c = 0; c < m; c++) {
for (d = 0; d < q; d++)
printf(“%d\t”, multiply[c][d]);
printf(“\n”);
}
}
return 0;
}
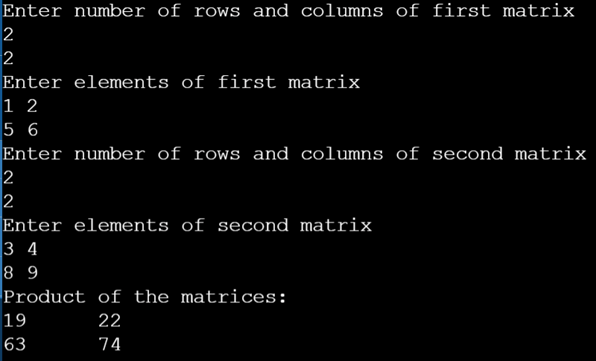
Displaying any part of a matrix
This operation is important when we need to display any part of a matrix-like the upper half or lower half. In these diagonals, elements play an important role and their position.
#include<stdio.h>
int main()
{
int i, j, row, column, arr[20][20];
printf(“\n Enter the Number of rows and columns : “);
scanf(“%d %d”, &i, &j);
printf(“\n Enter the Matrix Elements \n”);
for(row = 0; row < i; row++)
{
for(column = 0;column < j;column++)
{
scanf(“%d”, &arr[row][column]);
}
}
for(row = 0; row < i; row++)
{
printf(“\n”);
for(column = 0; column < j; column++)
{
if(column >= row)
{
printf(“%d “, arr[row][column]);
}
else
{
printf(“0 “);
}
}
}
return 0;
}

In the upcoming post, you will be getting information related to functions in c/c++ and Formal and actual parameters. If you haven’t read about What is a two-dimensional array then go and read this. Also, stay tuned for the upcoming posts. You can also refer programmiz.
Nice and informative content👍👍
amazing content 😊🙌🏻
amazing😊🙌🏻